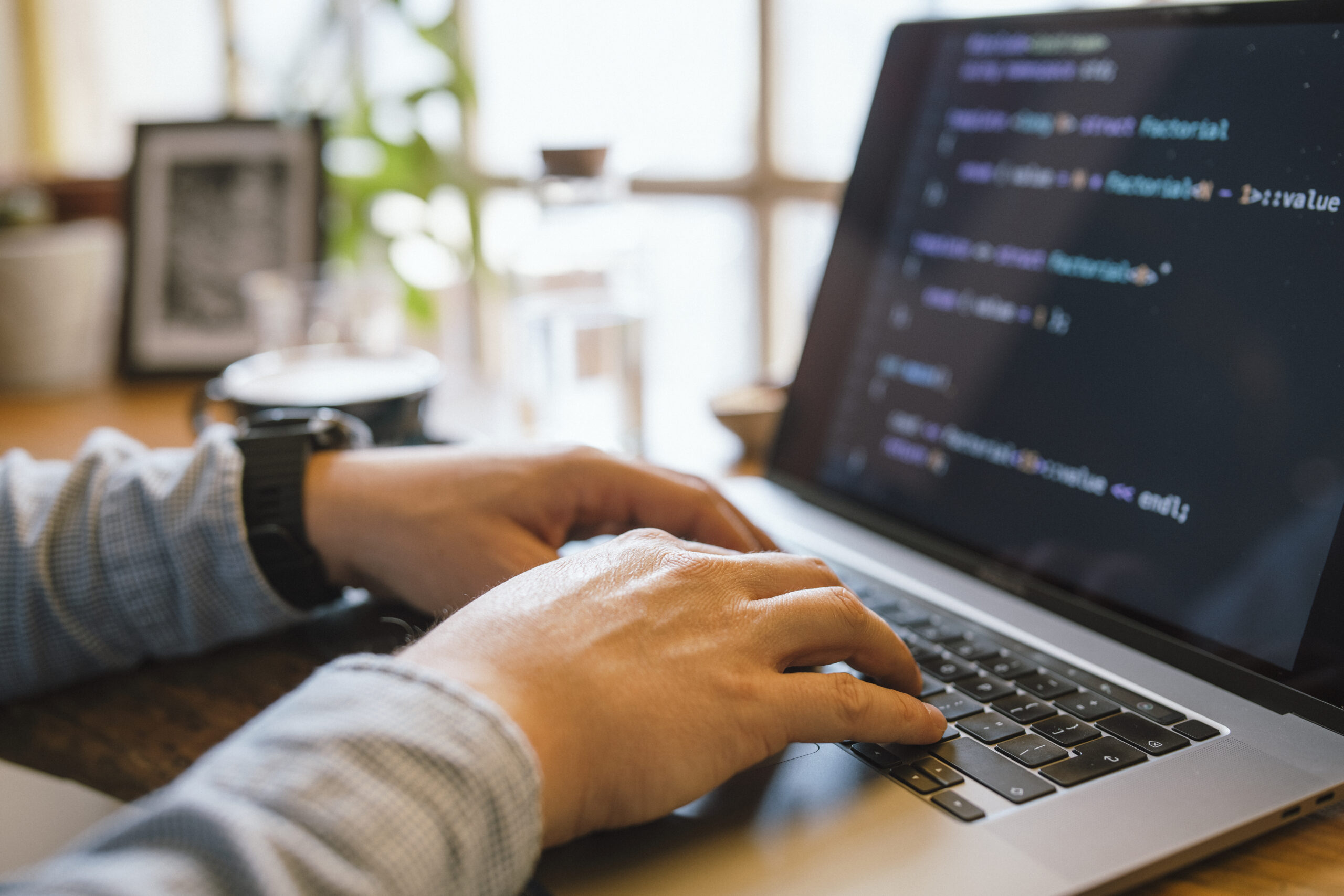
Debugging is The most essential — but generally missed — abilities inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why things go Incorrect, and Understanding to Consider methodically to resolve challenges successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of irritation and dramatically improve your efficiency. Here are quite a few procedures that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Resources
One of many quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person A part of development, understanding how to connect with it proficiently for the duration of execution is equally essential. Modern enhancement environments arrive equipped with powerful debugging abilities — but several builders only scratch the floor of what these resources can perform.
Just take, for instance, an Integrated Development Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, step by code line by line, and also modify code on the fly. When utilised properly, they Enable you to observe just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, keep an eye on community requests, check out genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can turn annoying UI issues into manageable jobs.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about operating processes and memory administration. Discovering these tools could have a steeper Mastering curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, turn out to be relaxed with Variation Command programs like Git to be aware of code record, find the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your applications means going past default settings and shortcuts — it’s about building an intimate understanding of your growth natural environment making sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most essential — and sometimes disregarded — measures in efficient debugging is reproducing the problem. Before leaping in the code or generating guesses, developers need to produce a regular setting or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a video game of possibility, frequently leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question concerns like: What steps resulted in The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it becomes to isolate the precise conditions underneath which the bug occurs.
When you finally’ve collected plenty of details, seek to recreate the trouble in your neighborhood surroundings. This could signify inputting exactly the same facts, simulating comparable person interactions, or mimicking system states. If The problem seems intermittently, think about creating automatic tests that replicate the edge scenarios or state transitions concerned. These assessments don't just aid expose the situation but also avoid regressions Down the road.
Occasionally, The problem can be environment-certain — it'd materialize only on particular working devices, browsers, or under distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. That has a reproducible state of affairs, You may use your debugging applications more properly, take a look at probable fixes safely and securely, and converse far more Plainly using your workforce or buyers. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Examine and Recognize the Error Messages
Error messages tend to be the most valuable clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, developers ought to learn to take care of mistake messages as direct communications from the procedure. They generally let you know precisely what happened, wherever it occurred, and occasionally even why it transpired — if you know the way to interpret them.
Start out by looking through the message diligently and in complete. Lots of developers, especially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — go through and understand them initially.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a particular file and line number? What module or operate brought on it? These queries can guideline your investigation and level you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Understanding to acknowledge these can drastically accelerate your debugging course of action.
Some errors are obscure or generic, As well as in Those people instances, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede bigger troubles and supply hints about opportunity bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them properly turns chaos into clarity, supporting you pinpoint challenges faster, decrease debugging time, and become a far more economical and self-assured developer.
Use Logging Properly
Logging is The most impressive tools within a developer’s debugging toolkit. When utilised effectively, it provides genuine-time insights into how an software behaves, serving to you have an understanding of what’s going on under the hood without needing to pause execution or step through the code line by line.
A good logging technique starts with knowing what to log and at what level. Popular logging concentrations involve DEBUG, Data, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic facts through progress, Data for basic activities (like productive begin-ups), Alert for probable troubles that don’t split the application, Mistake for real issues, and Lethal if the program can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your technique. Give attention to important events, point out adjustments, input/output values, and significant selection details as part of your code.
Format your log messages Evidently and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even much easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Specifically valuable in generation environments exactly where stepping as a result of code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, wise logging is about stability and clarity. Which has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly detect and repair bugs, developers have to solution the procedure like a detective solving a thriller. This frame of mind allows stop working complicated concerns into manageable sections and observe clues logically to uncover the foundation cause.
Begin by collecting evidence. Consider the indicators of the issue: error messages, incorrect output, or efficiency troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s happening.
Subsequent, sort hypotheses. Inquire by yourself: What may be triggering this habits? Have any alterations just lately been created towards the codebase? Has this difficulty happened in advance of underneath very similar situation? The intention should be to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Attempt to recreate the problem inside of a controlled atmosphere. If you suspect a selected operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay near interest to compact information. Bugs frequently disguise inside the the very least anticipated places—just like a missing semicolon, an off-by-one particular error, or maybe a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly knowing it. Non permanent fixes could disguise the real dilemma, just for it to resurface later.
And lastly, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other people fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate systems.
Compose Assessments
Crafting tests is one of the most effective approaches to transform your debugging competencies and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can promptly expose no matter if a certain piece of logic is Functioning as anticipated. Whenever a test fails, you immediately know where by to glimpse, noticeably cutting down enough time invested debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These help be sure that a variety of elements of your software get the job done collectively smoothly. They’re specially beneficial for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a function thoroughly, you may need to know its inputs, predicted outputs, and edge cases. This standard of comprehending Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails continuously, you'll be able to center on fixing the bug and observe your take a look at go when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from a annoying guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new perspective.
If you're too near the code for much too long, cognitive exhaustion sets in. You may perhaps start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the qualifications.
Breaks also assist prevent burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
For those who’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, each one can educate you anything precious if you make an effort to reflect and examine what went Mistaken.
Start out by inquiring you a few important concerns after the bug is settled: What induced it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots within your workflow or knowing and allow you to Create more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring troubles or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether more info it’s by way of a Slack message, a brief compose-up, or a quick awareness-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers will not be the ones who publish perfect code, but individuals who continuously understand from their mistakes.
In the long run, each bug you correct provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll occur away a smarter, far more able developer as a result of it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a far more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.